Abstract
Alice and Bob each created one problem for HackerRank. A reviewer rates the two challenges, awarding points on a scale from 1 to 100 for three categories: problem clarity, originality, and difficulty.
Compare the Triplets#
The rating for Alice’s challenge is the triplet
a = (a[0], a[1], a[2])
, and the rating for Bob’s challenge is
the triplet b = (b[0], b[1], b[2])
.
The task is to find their comparison points by comparing a[0]
with b[0]
,
a[1]
with b[1]
, and a[2]
with b[2]
.
If a[i] > b[i]
, then Alice is awarded 1 point.
If a[i] < b[i]
, then Bob is awarded 1 point.
If a[i] = b[i]
, then neither person receives a point.
Comparison points is the total points a person earned.
Given a
and b
, determine their respective comparison points.
Example#
a = [1, 2, 3]
b = [3, 2, 1]
For elements
0
, Bob is awarded a point becausea[0] < b[0]
.For elements
1
, there is equalitya[1] = b[1]
, no points are earned.Finally, for elements 2,
a[2] > b[2]
so Alice receives a point.
The return array is [1, 1]
with Alice’s score first and Bob’s second.
Function Description#
Complete the function compare_triplets
in the editor below.
compare_triplets
has the following parameter(s):
- var list(int) a[3]:
Alice’s challenge rating
- var list(int) b[3]:
Bob’s challenge rating
Return
- var list(int)[2]:
Alice’s score is in the first position, and Bob’s score is in the second.
Input Format#
The first line contains 3 space-separated integers, a[0]
, a[1]
, and a[2]
,
the respective values in triplet a
.
The second line contains 3 space-separated integers, b[0]
, b[1]
, and b[2]
,
the respective values in triplet b
.
Constraints#
\(1 ≤ a[i] ≤ 100\)ß
\(1 ≤ b[i] ≤ 100\)
Sample Input 0#
5 6 7
3 6 10
Sample Output 0#
1 1
Explanation 0#
In this example:
\(a = (a[0], a[1], a[2]) = (5, 6, 7)\)
\(b = (b[0], b[1], b[2]) = (3, 6, 10)\)
Now, let’s compare each individual score:
\(a[0] > b[0]\), so Alice receives \(1\) point.
\(a[1] = b[1]\), so nobody receives a point.
\(a[2] < a[2]\), so Bob receives \(1\) point.
Alice’s comparison score is \(1\), and Bob’s comparison score is \(1\). Thus, we return the array \([1, 1]\).
Sample Input 1#
17 28 30
99 16 8
Sample Output 1#
2 1
Explanation 1#
Comparing the \(0^{th}\) elements, \(17 < 99\) so Bob receives a point.
Comparing the \(1^{st}\) and \(2^{nd}\) elements, \(28 > 16\) and \(30 > 8\) so Alice receives two points.
The return array is \([2, 1]\).
Python Solution Docs#
This module contains a function to compare triplet values.
- class compare_the_triplets.ScoreCompare(a_list, b_list)#
Define a ScoreCompare object.
- Attribute list a:
An empty list.
- Attribute list b:
Another empty list.
- Attribute list scores:
A list with two values initialized to 0.
- compare(a_int, b_int)#
Compare values for two integers.
- Parameters:
a_int (int) – An integer value
b_int (int) – Another integer value
- report_score()#
Report the current score.
- compare_the_triplets.compare_triplets(a_trip, b_trip)#
Compare sets of triplets to provide a score.
- Parameters:
a_trip (list) – INTEGER_ARRAY a, contains 3 values
b_trip (list) – INTEGER_ARRAY b, contains 3 values
- Returns:
The function is expected to return an INTEGER_ARRAY with 2 values.
compare the triplets#
#!/usr/bin/env python3
"""This module contains a function to compare triplet values."""
import os
import pathlib
class ScoreCompare:
"""Define a ScoreCompare object.
:attribute list a: An empty list.
:attribute list b: Another empty list.
:attribute list scores: A list with two values initialized to 0.
"""
a = []
b = []
scores = [0, 0]
def __init__(self, a_list, b_list):
self.a = a_list
self.b = b_list
def compare(self, a_int, b_int):
"""Compare values for two integers.
:param int a_int: An integer value
:param int b_int: Another integer value
"""
if a_int > b_int:
self.scores[0] += 1
elif a_int < b_int:
self.scores[1] += 1
def report_score(self):
"""Report the current score."""
return self.scores
def compare_triplets(a_trip, b_trip):
"""Compare sets of triplets to provide a score.
:param list a_trip: INTEGER_ARRAY a, contains 3 values
:param list b_trip: INTEGER_ARRAY b, contains 3 values
:return: The function is expected to return an INTEGER_ARRAY with 2 values.
"""
sc_obj = ScoreCompare(a_trip, b_trip)
for index, value in enumerate(a_trip):
sc_obj.compare(value, b_trip[index])
return sc_obj.scores
if __name__ == '__main__':
a = list(map(int, input().rstrip().split()))
b = list(map(int, input().rstrip().split()))
result = compare_triplets(a, b)
fpath = pathlib.Path(os.environ.get('OUTPUT_PATH'))
with fpath.open('w', encoding='utf-8') as fp_handle:
fp_handle.write(' '.join(map(str, result)))
fp_handle.write('\n')
SCM#
Available in the related GitHub issue.
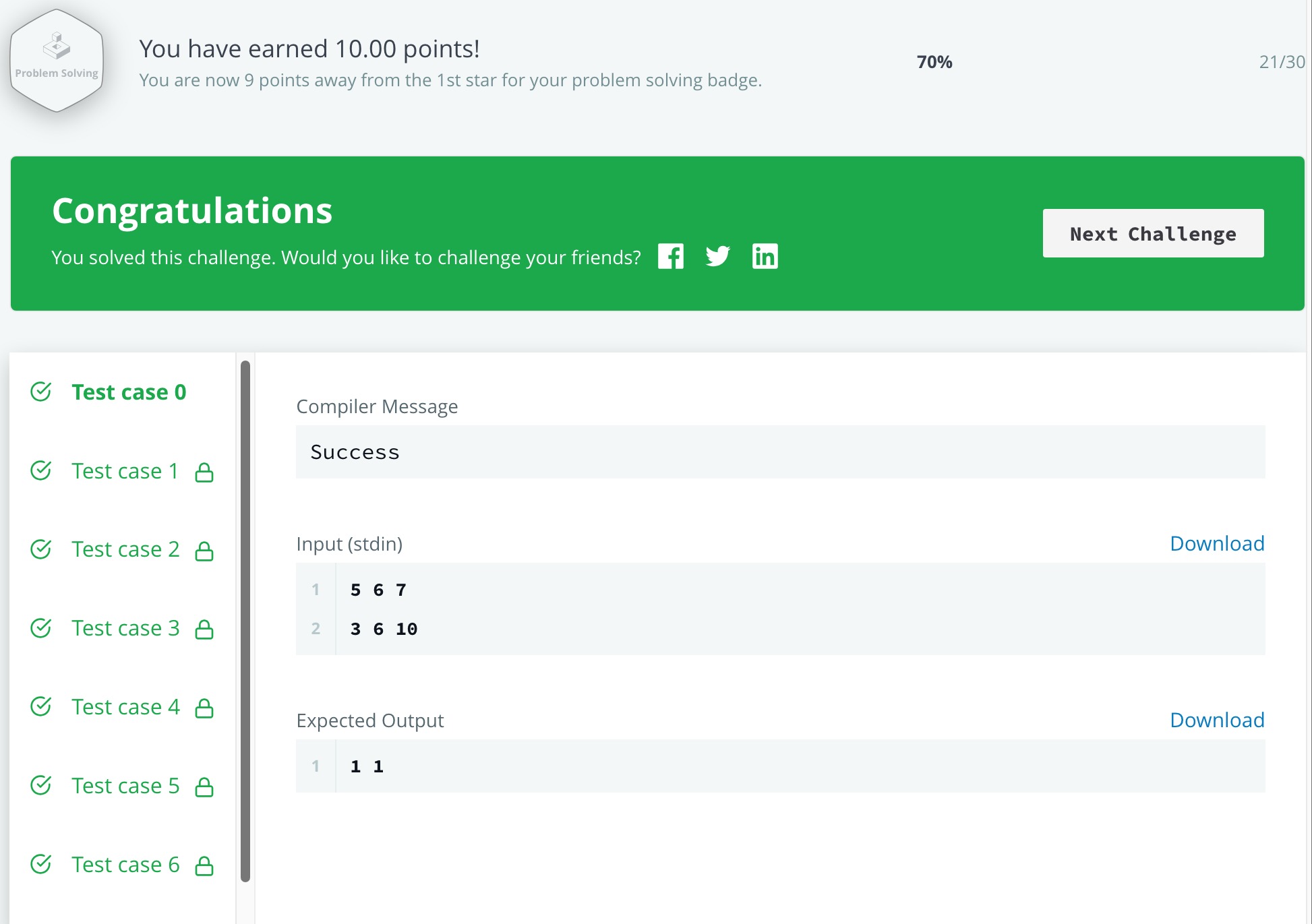
Section author: Xander Harris xandertheharris@gmail.com#