Abstract
Given an array of integers, find the sum of its elements.
Simple Array Sum#
Note
This was adapted from the Hacker Rank challenge available here.
Given an array of integers, find the sum of its elements.
For example, if the array \(ar = [1,2,3]\), \(1 + 2 + 3 = 6\), so return \(6\).
Function Description#
Complete the simple_array_sum function in the editor below. It must return the sum of the array elements as an integer.
simple_array_sum
has the following parameter(s):
list(ar)
an array of integers
Input Format#
The first line contains an integer, \(n\), denoting the size of the array. The second line contains \(n\) space-separated integers representing the array’s elements.
Constraints#
\(0<N,ar[i]\le1000\)
Output Format#
Print the sum of the array’s elements as a single integer.
Sample Input#
6
1 2 3 4 10 11
Sample Output#
31
Explanation#
We print the sum of the array’s elements: \(1 + 2 + 3 + 4 + 10 + 11 = 31\).
Python Solution Docs#
A simple module that prints the sum of an array of integers.
Assignment
— Complete the simple_array_sum function below.
The function is expected to return an INTEGER. The function accepts INTEGER_ARRAY ar as parameter.
- simple_array_sum.simple_array_sum(ar_list)#
Sum the contents of an array.
- Parameters:
ar_list (list) – A list of integers to sum.
simple array sum#
#!/usr/bin/env python3
"""
A simple module that prints the sum of an array of integers.
.. rubric:: Assignment
---
Complete the `simple_array_sum` function below.
The function is expected to return an `INTEGER`.
The function accepts `INTEGER_ARRAY` ar as parameter.
"""
import pathlib
import os
def simple_array_sum(ar_list):
"""Sum the contents of an array.
:param list ar_list: A list of integers to sum.
"""
try:
ret_value = sum(ar_list)
except TypeError as type_error:
raise TypeError("Please input only numeric values.") from type_error
return ret_value
if __name__ == '__main__':
ar_count = int(input().strip())
ar = list(map(int, input().rstrip().split()))
result = simple_array_sum(ar)
fpath = pathlib.Path(os.environ.get('OUTPUT_PATH'))
with fpath.open('w', encoding='utf-8') as f_handle:
f_handle.write(str(result) + '\n')
SCM#
Available in the related GitHub issue.
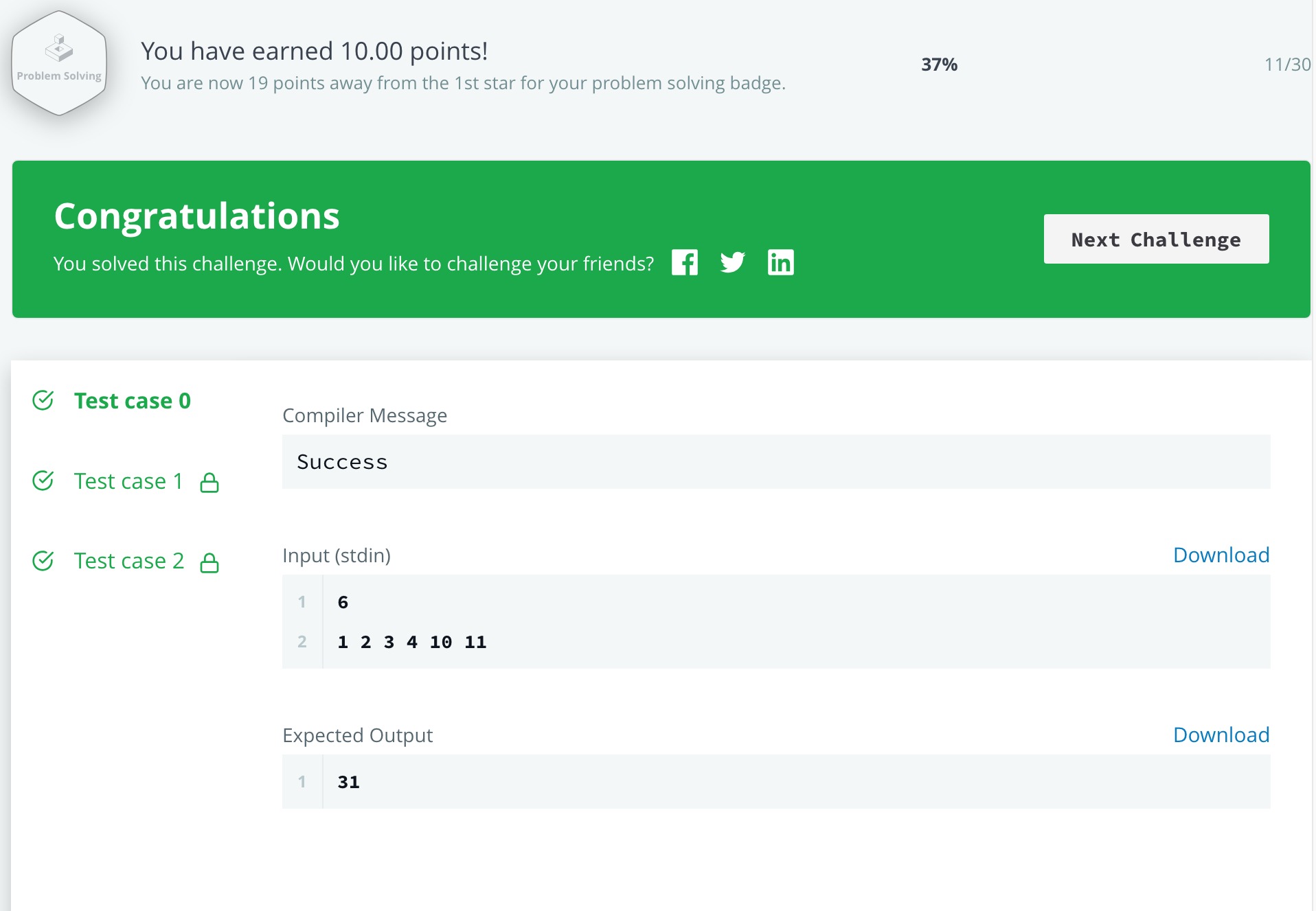
Section author: Xander Harris xandertheharris@gmail.com#